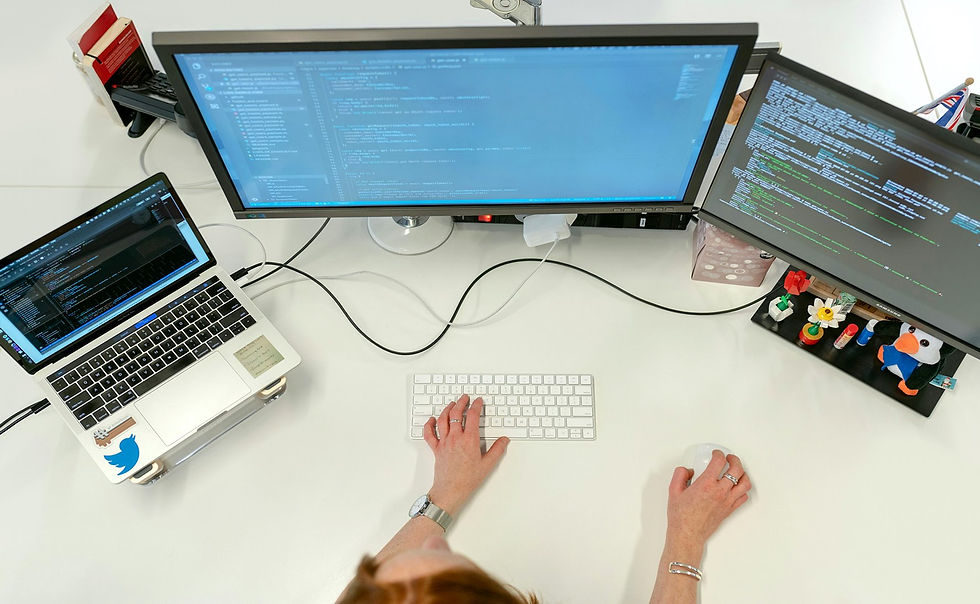
Dynamic Arrays in C++ | A Deep Dive into Vectors
Introduction
In C++, vectors are part of the Standard Template Library (STL) and serve as dynamic arrays that can resize themselves automatically when an element is added or removed. This flexibility makes vectors an essential tool in modern C++ programming. Unlike traditional arrays, which require a predefined size, vectors can expand and shrink as needed, making them ideal for situations where the number of elements isn't known in advance.
In this blog, we will explore what C++ vectors are, how they work, and the operations you can perform on them.
What is a C++ Vector?
A vector in C++ is a sequence container that can store elements of the same data type. It operates like an array but with the added advantage of dynamic resizing. Vectors use contiguous memory storage, which allows for efficient element access and iteration.
Here is the basic syntax for declaring a vector:

In this example, "myVector" is a vector of integers. You can also initialize vectors with specific values:

Key Features of C++ Vectors
Dynamic Resizing: Vectors automatically resize as elements are added or removed. This is achieved by allocating additional memory when the vector reaches its current capacity.
Efficient Element Access: Since vectors store elements in contiguous memory, accessing elements using an index (like an array) is very efficient.
Iterators: Vectors provide iterators that allow you to traverse and manipulate elements efficiently. Iterators behave like pointers and can be incremented or decremented to move through the vector.
STL Integration: Being part of the STL, vectors have access to a wide range of algorithms, including sorting, searching, and manipulating elements.
Operations on Vectors
1. Adding Elements to a Vector
You can add elements to a vector using the "push_back()" method. This method adds an element to the end of the vector:

2. Removing Elements from a Vector
To remove an element from a vector, you can use the "pop_back()" method, which removes the last element:

You can also remove elements at specific positions using the erase() method:

3. Accessing Elements
Elements in a vector can be accessed using the [] operator or the at() method:

4. Size and Capacity
Vectors provide the size() method to get the number of elements in the vector and the capacity() method to get the current storage capacity:

5. Inserting Elements
You can insert elements at any position in a vector using the insert() method:

6. Clearing the Vector
If you want to remove all elements from a vector, you can use the clear() method:

7. Checking if a Vector is Empty
To check if a vector is empty, you can use the empty() method:

Advantages of Using C++ Vectors
Dynamic Sizing:
Unlike arrays, vectors do not require a predefined size, making them highly flexible for varying data sizes.
Efficient Memory Management:
Vectors manage memory automatically, reducing the chances of memory leaks or buffer overflows.
Rich Functionality:
Vectors come with a variety of built-in functions and methods for inserting, deleting, and manipulating data.
When to Use Vectors
Vectors are ideal when you need:
Dynamic Arrays: If you expect the size of your data structure to change frequently, vectors are more convenient than arrays.
Efficient Iteration: With iterators, vectors provide efficient ways to iterate through elements.
STL Algorithms: Vectors work seamlessly with STL algorithms, such as sorting, searching, and filtering.
However, there are scenarios where vectors may not be the best choice. For example, if you need to insert or delete elements frequently at the beginning or middle of a container, "std::list" might be more efficient as it doesn't require shifting elements like vectors do.
Vector vs. Array in C++
Dynamic Size
Vector: Resizes automatically as elements are added or removed.
Array: Fixed size. You must declare the size at the time of initialization.
Memory Allocation
Vector: Allocates additional memory to allow for growth, which can result in some overhead.
Array: Allocates a fixed amount of memory, resulting in more predictable performance.
Functionality
Vector: Comes with built-in methods for adding, removing, and manipulating elements.
Array: Limited functionality. You have to manually manage memory and operations.
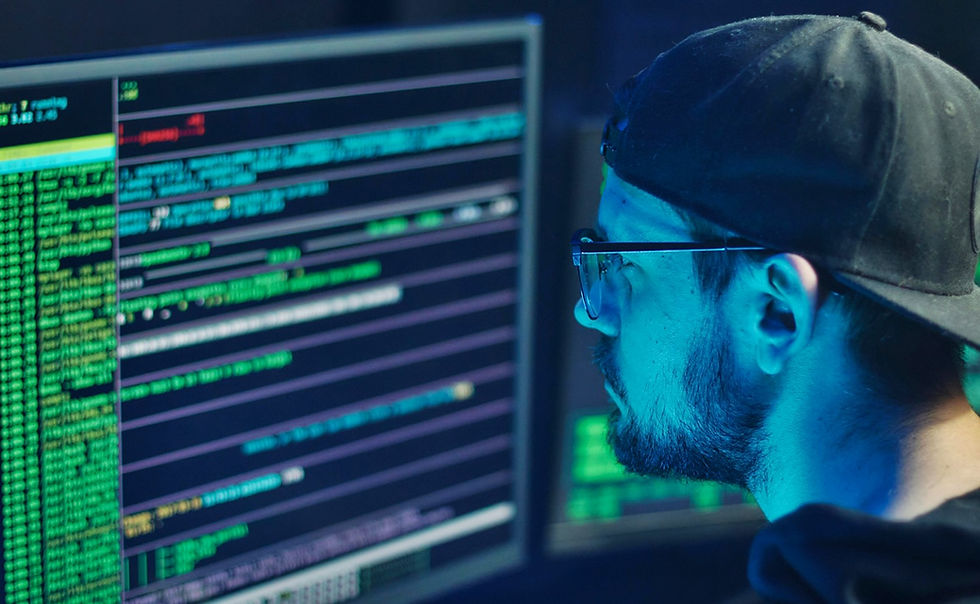
Conclusion | C++ Vectors
C++ vectors are a powerful and flexible alternative to arrays, offering dynamic sizing, efficient element access, and integration with STL algorithms. Whether you're working with a small dataset or need to handle large amounts of data that change dynamically, vectors provide a versatile solution.
By understanding how to use vectors effectively, you can write more efficient and maintainable C++ code. Whether you're adding elements, iterating through a list, or integrating with STL algorithms, vectors offer a convenient and powerful tool for your programming needs.
c++ vector, c++ vector initialization, c++ vector erase, c++ vector find, c++ vector sort, c++ vector insert, c++ vector push_back, c++ vector functions, c++ vector vs array, c++ vector contains, c++ vector resize, c++ vector clear, c++ vector methods, c++ vector example, c++ vector length, sort function in c++ vector, accumulate c++ vector, max_element c++ vector
Comments